.netCHARTING Data Classes
The .netCHARTING data model consists of elements which collectively become a series which can be added to a SeriesCollection.
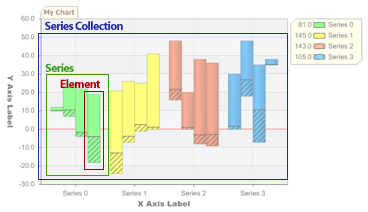
Data Class |
Description |
---|---|
Element |
Represents an element on the chart and facilitates the following.
|
Series |
Utilizes the ElementCollection class to hold a set of elements and facilitates the following.
|
SeriesCollection |
Contains a collection of series and facilitates the following.
|
ElementCollection | Used by the Series class to hold a collection of elements. |
Using Data Classes
The following example creates a SeriesCollection containing one Series which contains a single Element using the following steps.
-
Instantiates an element and sets it's properties.
-
Instantiates a series and adds the element to it.
-
Instantiates a SeriesCollection and adds the series to it.
[C#] Element e = new Element(); // Instantiate the element e.Name = "Element 1"; // Name it e.YValue = 10; // Set the y value Series s = new Series(); // Instantiate the series s.Name = "Series 1"; // Name it s.Elements.Add(e); // Add element e to series s. SeriesCollection sc = new SeriesCollection(); // Instantiate a series collection sc.Add(s); // Add series s to the series collection sc. //The sc object now contains data we can chart which we add here. Chart.SeriesCollection.Add(sc);
[Visual Basic] Dim e As New Element() ' Instantiate the element e.Name = "Element 1" ' Name it e.YValue = 10 ' Set the y value Dim s As New Series() ' Instantiate the series s.Name = "Series 1" ' Name it s.Elements.Add(e) ' Add element e to series s. Dim sc As New SeriesCollection() ' Instantiate a series collection sc.Add(s) ' Add series s to the series collection sc. 'The sc object now contains data we can chart which we add here. Chart.SeriesCollection.Add(sc)
Shortcuts
.netCHARTING provides many shortcuts which can make adding data easier. The previous example can also be achieved using the following shortcuts.
Using Chart.Series shortcut.
[C#] Element e = new Element(); e.Name = "Element 1"; e.YValue = 10; Chart.Series.Add(e); Chart.SeriesCollection.Add(); // Not passing parameters will add the contents of Chart.Series to the Chart.SeriesCollection collection.
[Visual Basic] Dim e As New Element() e.Name = "Element 1" e.YValue = 10 Chart.Series.Add(e) Chart.SeriesCollection.Add() ' Not passing parameters will add the contents of Chart.Series to the Chart.SeriesCollection collection.
Using the Chart.Element shortcut.
[C#] Chart.Series.Element.YValue = 10; Chart.Series.Element.Name = "Element 1"; Chart.Series.Elements.Add(); Chart.SeriesCollection.Add();
[Visual Basic] Chart.Series.Element.YValue = 10 Chart.Series.Element.Name = "Element 1" Chart.Series.Elements.Add() Chart.SeriesCollection.Add()
Using all the shortcuts allows us to reduce the amount of lines necessary to achieve the same result from 8 to 4. The down side is that after the data is added using Add(), it cannot be retrieved for further manipulation.
Take it to the next step
It is still possible to reduce the code even further using element constructors.
[C#] Chart.SeriesCollection.Add(new Element("Element1",10));
[Visual Basic] Chart.SeriesCollection.Add(New Element("Element1",10))
Now we took it from 8 lines to 1.
For more information on code shortcuts, see the Efficient Code tutorial.